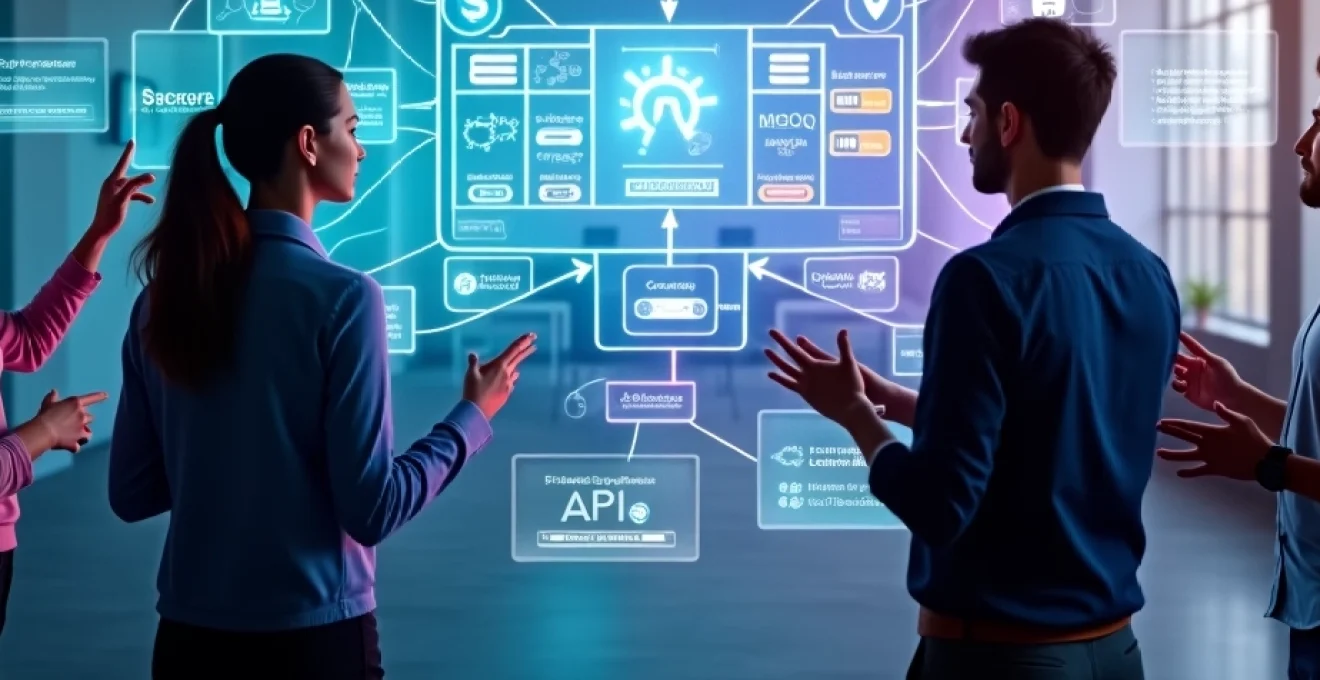
Application Programming Interfaces (APIs) have become the backbone of modern web development, revolutionizing how applications communicate and share data. As the digital landscape evolves, APIs play an increasingly crucial role in creating seamless, efficient, and scalable web applications. They enable developers to integrate complex functionalities, access third-party services, and build robust, interconnected systems without reinventing the wheel.
The importance of APIs in today's web development ecosystem cannot be overstated. They serve as the connective tissue between different software components, allowing for modular development, improved performance, and enhanced user experiences. From simple data retrieval to complex microservices architectures, APIs are essential for building flexible, maintainable, and future-proof web applications.
RESTful API architecture for web services
RESTful (Representational State Transfer) APIs have become the de facto standard for web services due to their simplicity, scalability, and stateless nature. REST APIs use standard HTTP methods like GET, POST, PUT, and DELETE to perform operations on resources, making them intuitive for developers and easy to implement across various platforms.
One of the key advantages of RESTful APIs is their ability to leverage existing web infrastructure, including caching mechanisms and security protocols. This makes them highly efficient for web applications that require frequent data exchanges between clients and servers. Additionally, REST APIs are language-agnostic, allowing developers to build clients and servers in different programming languages while maintaining compatibility.
When designing RESTful APIs, it's crucial to follow best practices such as using meaningful resource names, implementing proper status codes, and ensuring proper versioning. These practices contribute to creating APIs that are not only functional but also developer-friendly and easy to maintain over time.
GraphQL: flexible data querying in modern web apps
While REST has been the dominant API paradigm for years, GraphQL has emerged as a powerful alternative, particularly for complex applications with diverse data requirements. Developed by Facebook, GraphQL offers a more flexible and efficient approach to data fetching and manipulation.
Unlike REST, which typically requires multiple endpoints for different resources, GraphQL provides a single endpoint where clients can request exactly the data they need. This approach significantly reduces over-fetching and under-fetching of data, leading to more efficient network usage and improved application performance.
Schema definition language (SDL) in GraphQL
At the heart of GraphQL is its Schema Definition Language (SDL), which allows developers to define the structure of their API in a clear, language-agnostic way. The SDL describes the types of data available through the API, the relationships between these types, and the operations that can be performed.
Using SDL, developers can create a contract between the client and server, ensuring that both sides have a clear understanding of the data structure and available operations. This schema-first approach promotes better collaboration between front-end and back-end teams, as well as improved API documentation.
Resolvers and data fetching mechanisms
In GraphQL, resolvers are functions that define how to fetch or compute the data for each field in the schema. These resolvers provide the flexibility to retrieve data from various sources, including databases, other APIs, or even computed values.
The resolver-based approach allows for efficient data fetching, as GraphQL can execute multiple resolvers in parallel, optimizing the overall query performance. This is particularly beneficial for complex queries that require data from multiple sources or have deeply nested relationships.
Apollo client for front-end integration
Apollo Client has emerged as a popular choice for integrating GraphQL into front-end applications. It provides a comprehensive set of tools and features that simplify state management, caching, and error handling when working with GraphQL APIs.
With Apollo Client, developers can easily manage local and remote data, implement optimistic UI updates, and handle complex data synchronization scenarios. Its integration with popular front-end frameworks like React, Vue, and Angular makes it a versatile choice for modern web development projects.
Graphql subscriptions for real-time updates
One of the powerful features of GraphQL is its support for real-time updates through subscriptions. Unlike traditional request-response models, subscriptions allow clients to receive continuous updates from the server as data changes.
This capability is particularly useful for building real-time features such as live chat applications, collaborative tools, or any scenario where immediate data synchronization is crucial. GraphQL subscriptions provide a more efficient alternative to polling or WebSocket-based solutions for real-time updates.
Microservices and API gateways
The rise of microservices architecture has further emphasized the importance of APIs in modern web development. Microservices rely heavily on APIs for inter-service communication, allowing developers to build complex applications as a collection of loosely coupled, independently deployable services.
In a microservices ecosystem, API gateways play a crucial role in managing and orchestrating requests between clients and the various microservices. They act as a single entry point for all client requests, handling tasks such as request routing, authentication, rate limiting, and protocol translation.
Kong API gateway for traffic management
Kong is a popular open-source API gateway that offers robust features for managing API traffic in microservices architectures. It provides capabilities such as load balancing, caching, authentication, and analytics, making it easier to scale and manage complex API ecosystems.
With Kong, developers can implement advanced traffic control policies, monitor API usage, and ensure high availability of their services. Its plugin architecture allows for easy extensibility, enabling teams to add custom functionality or integrate with existing tools and workflows.
Oauth 2.0 and JWT for API authentication
Security is a critical concern in API development, and OAuth 2.0 has become the industry standard for secure API authentication and authorization. Combined with JSON Web Tokens (JWT), OAuth 2.0 provides a flexible and scalable solution for managing access to API resources.
OAuth 2.0 allows applications to obtain limited access to user accounts on an HTTP service, typically through access tokens. JWTs, on the other hand, are compact, self-contained tokens that can securely transmit information between parties as a JSON object.
Implementing OAuth 2.0 and JWT in API authentication flows ensures that only authorized clients can access protected resources, while also providing a seamless user experience through features like single sign-on (SSO) and token refresh mechanisms.
API versioning strategies
As APIs evolve over time, versioning becomes crucial to maintain backward compatibility and manage changes effectively. There are several strategies for API versioning, each with its own advantages and trade-offs:
- URL versioning: Including the version number in the API endpoint URL
- Header versioning: Specifying the desired version in the request headers
- Query parameter versioning: Adding the version as a query parameter in the request
- Content negotiation: Using Accept headers to specify the desired API version
Choosing the right versioning strategy depends on factors such as the API's complexity, client requirements, and long-term maintainability goals. Regardless of the chosen approach, clear communication and documentation of versioning policies are essential for API consumers.
Websocket APIs for real-time communication
While HTTP-based APIs are suitable for most web applications, some scenarios require real-time, bidirectional communication between clients and servers. WebSocket APIs fill this gap by providing a full-duplex, low-latency communication channel over a single TCP connection.
WebSockets are particularly useful for applications that require instant updates, such as chat applications, live sports scores, or collaborative tools. Unlike traditional HTTP requests, WebSocket connections remain open, allowing servers to push data to clients without the need for polling or long-polling techniques.
Implementing WebSocket APIs requires careful consideration of factors such as connection management, message formatting, and scalability. Libraries like Socket.IO have simplified WebSocket implementation by providing fallback mechanisms and additional features like room-based broadcasting and automatic reconnection.
API documentation with OpenAPI (swagger)
Comprehensive and up-to-date documentation is crucial for the success of any API. The OpenAPI Specification (formerly known as Swagger) has emerged as the industry standard for describing RESTful APIs, providing a language-agnostic way to document API endpoints, request/response formats, and authentication requirements.
OpenAPI documentation not only serves as a reference for API consumers but also facilitates API-first development practices, where the API design drives the implementation process. This approach promotes better planning, consistency, and overall API quality.
Swagger UI for interactive API exploration
Swagger UI is a popular tool that generates interactive documentation from OpenAPI specifications. It provides a user-friendly interface for exploring API endpoints, making test requests, and visualizing response data.
By integrating Swagger UI into their development workflow, teams can create living documentation that stays synchronized with the actual API implementation. This reduces the likelihood of documentation becoming outdated and improves the overall developer experience for API consumers.
API blueprint for machine-readable documentation
API Blueprint is another popular format for creating machine-readable API documentation. It uses a Markdown-based syntax to describe API endpoints, data structures, and example requests/responses.
One of the advantages of API Blueprint is its simplicity and readability, making it accessible to both technical and non-technical stakeholders. Tools like Apiary can generate interactive documentation and mock servers from API Blueprint specifications, facilitating rapid prototyping and collaboration.
Postman collections for API testing
Postman has become an indispensable tool for API development and testing. Its Collections feature allows developers to group related API requests, making it easier to organize, share, and automate API tests.
Postman Collections can be exported and shared with team members or API consumers, providing a practical way to demonstrate API usage and streamline the onboarding process. Additionally, Postman's scripting capabilities enable the creation of complex test scenarios and environment-specific configurations.
API performance optimization techniques
As APIs become central to web applications, optimizing their performance is crucial for ensuring a smooth user experience and efficient resource utilization. Several techniques can be employed to enhance API performance:
Caching strategies with Redis
Implementing effective caching mechanisms can significantly reduce the load on backend services and improve API response times. Redis, an in-memory data structure store, is widely used for caching API responses and frequently accessed data.
By caching API responses at various levels (application, database, or CDN), developers can reduce the number of expensive computations or database queries, leading to faster response times and improved scalability. However, it's important to implement proper cache invalidation strategies to ensure data consistency.
Rate limiting and throttling implementation
Rate limiting is essential for protecting APIs from abuse and ensuring fair usage among clients. By implementing rate limiting, developers can control the number of requests a client can make within a specified time frame, preventing server overload and maintaining service quality for all users.
Various algorithms can be used for rate limiting, such as the token bucket or leaky bucket algorithms. Additionally, implementing throttling mechanisms can help manage traffic spikes by gradually reducing the rate of accepted requests as the system approaches its capacity limits.
Compression and minification for payload reduction
Reducing the size of API payloads can lead to significant performance improvements, especially for bandwidth-constrained environments. Implementing compression algorithms like gzip for HTTP responses can substantially reduce the amount of data transferred over the network.
For APIs that return large JSON payloads, minification techniques can be applied to remove unnecessary whitespace and reduce the overall response size. However, it's important to balance payload reduction with readability, especially for APIs that are consumed directly by developers.
Load balancing with NGINX
Load balancing is crucial for distributing API traffic across multiple server instances, ensuring high availability and optimal resource utilization. NGINX, a popular web server and reverse proxy, offers robust load balancing capabilities for API deployments.
NGINX can be configured to use various load balancing algorithms, such as round-robin, least connections, or IP hash, depending on the specific requirements of the API. Additionally, NGINX's caching and SSL termination features can further enhance API performance and security.
By implementing these performance optimization techniques, developers can create APIs that are not only functional but also highly efficient and scalable. Regular performance monitoring and optimization should be an ongoing process to ensure that APIs continue to meet the evolving needs of their consumers and maintain high standards of reliability and responsiveness.