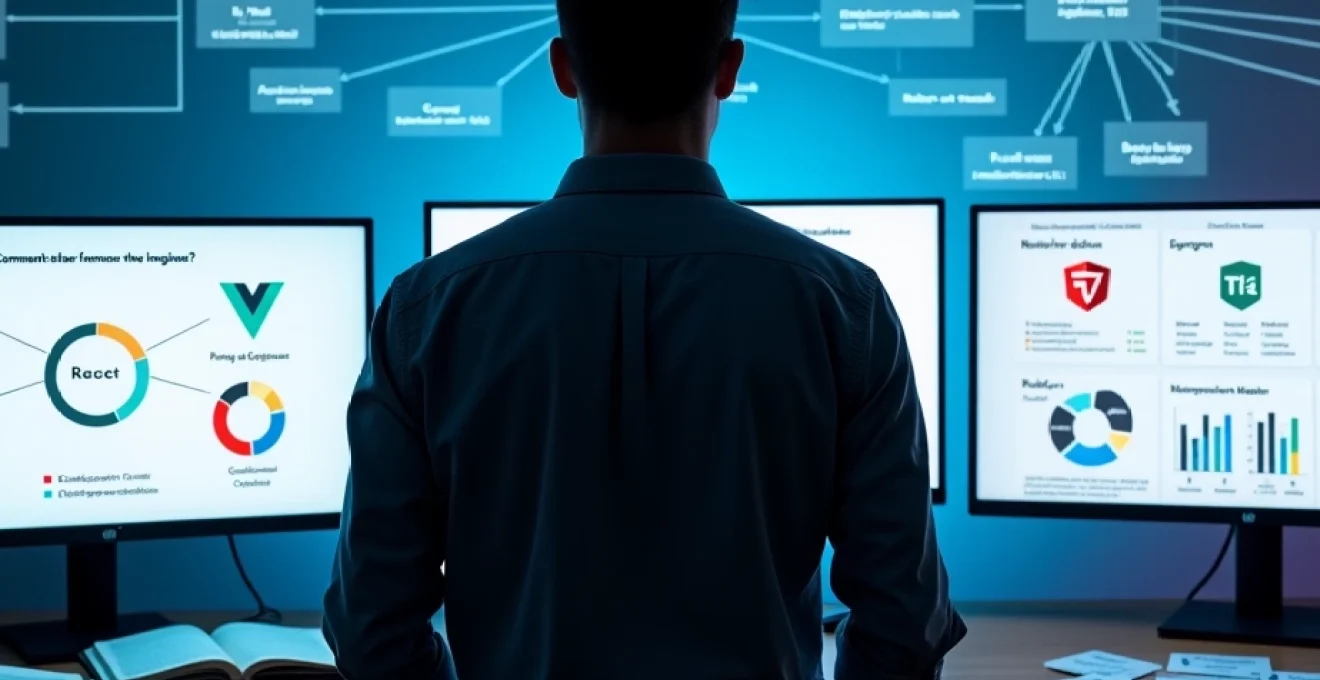
Selecting the right web framework is a crucial decision that can significantly impact the success of your project. With the rapid evolution of web technologies, developers are faced with an array of options, each offering unique features and advantages. This comprehensive guide will explore the key factors to consider when choosing a modern web framework, helping you make an informed decision that aligns with your project requirements and development goals.
Evaluating frontend frameworks: React, Vue, and Angular
When it comes to frontend development, three frameworks stand out as the most popular choices: React, Vue, and Angular. Each of these frameworks has its strengths and use cases, making the selection process a matter of aligning your project needs with the framework's capabilities.
React, developed by Facebook, is known for its component-based architecture and virtual DOM, which enable efficient rendering and updates. It's particularly well-suited for large-scale applications and offers a rich ecosystem of libraries and tools. Vue, on the other hand, is praised for its simplicity and gentle learning curve, making it an excellent choice for smaller projects or teams new to frontend frameworks.
Angular, maintained by Google, provides a complete solution for building complex applications. It includes features like two-way data binding and dependency injection out of the box, which can significantly speed up development for enterprise-level projects. However, it also comes with a steeper learning curve compared to React and Vue.
When evaluating these frameworks, consider factors such as:
- The size and complexity of your project
- Your team's expertise and learning capacity
- Performance requirements
- Long-term maintainability
- Community support and available resources
Backend considerations: Node.js, Django, and Ruby on Rails
Choosing the right backend framework is equally important as it forms the foundation of your web application's server-side logic. Let's explore three popular options: Node.js, Django, and Ruby on Rails.
Node.js and Express: JavaScript Full-Stack development
Node.js, coupled with the Express framework, has gained significant popularity due to its ability to use JavaScript on both the frontend and backend. This allows for full-stack JavaScript development, which can streamline the development process and reduce context switching for developers.
Node.js excels in building real-time applications, APIs, and microservices. Its event-driven, non-blocking I/O model makes it highly efficient for handling concurrent connections, making it an excellent choice for scalable applications. However, it may not be the best fit for CPU-intensive tasks or applications requiring complex database operations.
Django: Python-powered web applications
Django is a high-level Python web framework that follows the "batteries included" philosophy, providing a comprehensive set of tools and features out of the box. It's known for its robust ORM (Object-Relational Mapping), built-in admin interface, and strong security measures.
Django is particularly well-suited for content-heavy websites, complex database-driven applications, and projects that require rapid development. Its emphasis on the DRY (Don't Repeat Yourself) principle and automatic admin interface generation can significantly speed up development time. However, this comprehensiveness can sometimes lead to a steeper learning curve for beginners.
Ruby on Rails: rapid development with convention over configuration
Ruby on Rails, often referred to as Rails, is known for its convention over configuration approach, which can dramatically reduce the amount of code needed to build web applications. This makes it an excellent choice for startups and projects that require rapid prototyping and iteration.
Rails shines in creating database-backed web applications and follows the MVC (Model-View-Controller) pattern. It comes with a rich set of helper methods and tools that can boost productivity. However, it may face performance challenges when scaling to very large applications, and its opinionated nature can sometimes be limiting for highly customized projects.
Full-stack frameworks: Next.js, Nuxt.js, and Nest.js
Full-stack frameworks have gained popularity by offering integrated solutions for both frontend and backend development. These frameworks aim to simplify the development process by providing a unified approach to building web applications.
Next.js, built on top of React, offers server-side rendering, static site generation, and API routes out of the box. It's particularly well-suited for building SEO-friendly React applications and can significantly improve performance through optimized rendering strategies.
Nuxt.js provides similar benefits for Vue.js applications, offering server-side rendering and static site generation capabilities. It also includes features like automatic code splitting and asynchronous data fetching, which can enhance the performance and user experience of Vue applications.
Nest.js, on the other hand, is a TypeScript-based framework for building efficient and scalable server-side applications. It leverages modern JavaScript features and design patterns, making it an excellent choice for developers looking to build robust backend services with a structure similar to Angular.
Performance metrics: Lighthouse Scores and Web Vitals
When evaluating web frameworks, it's crucial to consider their impact on performance metrics, particularly Lighthouse scores and Web Vitals. These metrics provide valuable insights into how well your application performs in terms of speed, accessibility, and user experience.
First Contentful Paint (FCP) optimization
First Contentful Paint (FCP) measures the time it takes for the first piece of content to appear on the screen. Optimizing FCP is crucial for providing a responsive feel to your web application. Frameworks that support server-side rendering or static site generation, like Next.js or Gatsby, can significantly improve FCP by delivering pre-rendered content to the browser.
Time to Interactive (TTI) improvement strategies
Time to Interactive (TTI) measures how long it takes for a page to become fully interactive. Frameworks that support code splitting and lazy loading, such as React with its React.lazy()
function, can help improve TTI by reducing the initial JavaScript payload and loading components only when needed.
Cumulative Layout Shift (CLS) minimization techniques
Cumulative Layout Shift (CLS) measures visual stability by quantifying unexpected layout shifts during page load. Frameworks that provide robust styling solutions and responsive design patterns can help minimize CLS. For example, CSS-in-JS solutions like styled-components in React or Vuetify in Vue.js can help create more predictable layouts.
When choosing a framework, consider how it supports these performance optimizations out of the box and how easily you can implement additional optimizations if needed.
Scalability and microservices architecture
As your application grows, scalability becomes a critical concern. Modern web frameworks should support scalable architectures, particularly microservices, which allow for better resource allocation and easier maintenance of large-scale applications.
Node.js, with its event-driven architecture, is particularly well-suited for building microservices. Frameworks like Express.js or Nest.js can be used to create lightweight, independent services that communicate via APIs. This approach allows for easier scaling of individual components and better fault isolation.
For frontend frameworks, consider how well they support code splitting and lazy loading. React's ecosystem, for instance, offers tools like Create React App that come with built-in code splitting capabilities, allowing you to load only the necessary components for each route.
When evaluating frameworks for scalability, ask yourself:
- How well does the framework support modular architecture?
- Can it easily integrate with containerization technologies like Docker?
- Does it provide tools for monitoring and managing distributed systems?
- How does it handle state management in large-scale applications?
Developer experience: TypeScript integration and hot module replacement
The developer experience provided by a framework can significantly impact productivity and code quality. Two key features to consider are TypeScript integration and Hot Module Replacement (HMR).
TypeScript: static typing for JavaScript ecosystems
TypeScript has become increasingly popular in modern web development due to its ability to add static typing to JavaScript. This can lead to improved code quality, better tooling support, and easier refactoring. When choosing a framework, consider how well it supports TypeScript out of the box.
Angular, for instance, is built with TypeScript in mind and provides excellent integration. React and Vue also offer strong TypeScript support, with Vue 3 being rewritten in TypeScript to provide better type inference.
Webpack and Vite: comparing build tools
Build tools play a crucial role in modern web development, with Webpack being a popular choice for many frameworks. However, newer tools like Vite are gaining traction due to their faster build times and improved developer experience.
Vite, developed by the creator of Vue.js, offers near-instantaneous server start and hot module replacement. It's framework-agnostic and can be used with React, Vue, or vanilla JavaScript projects. When evaluating frameworks, consider whether they support or recommend specific build tools and how these tools impact development speed and ease of use.
State management: Redux, Vuex, and NgRx
For complex applications, state management becomes a crucial consideration. Different frameworks have their preferred state management solutions:
- React often uses Redux or MobX
- Vue has Vuex (or Pinia for Vue 3)
- Angular commonly uses NgRx
When choosing a framework, consider the complexity of your application's state and how well the framework's ecosystem supports state management. Look for solutions that provide features like time-travel debugging, easy integration with developer tools, and support for asynchronous actions.
Remember, the best framework for your project depends on a combination of factors including your team's expertise, project requirements, and long-term maintenance considerations. By carefully evaluating these aspects, you can choose a modern web framework that not only meets your current needs but also supports your application's growth and evolution over time.