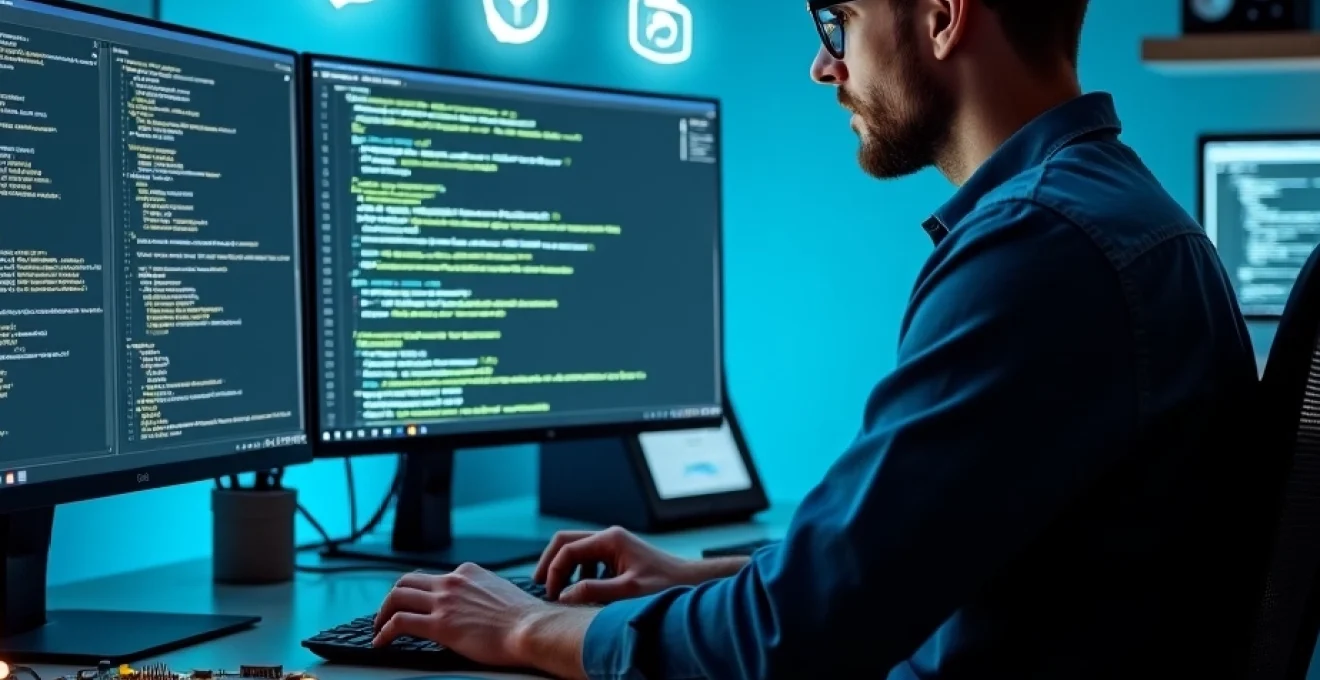
Microcontroller software development presents unique challenges that differ significantly from traditional desktop or mobile programming. These resource-constrained devices demand highly efficient code to deliver optimal performance while managing limited processing power, memory, and energy consumption. Creating high-performance software for microcontrollers requires specialized knowledge of hardware architectures, memory management techniques, and optimization strategies tailored to these small yet powerful computing platforms.
The landscape of microcontroller applications continues to expand rapidly, from smart home devices and wearable technology to industrial automation systems and automotive controls. As these applications grow in complexity, developers must balance functional requirements with the inherent limitations of microcontroller hardware. This balance becomes even more critical as Internet of Things (IoT) deployments accelerate, placing greater demands on these tiny computing platforms.
Modern microcontrollers offer sophisticated features like advanced timers, direct memory access controllers, and specialized hardware accelerators that, when properly leveraged, can dramatically improve application performance. Understanding how to utilize these features effectively is essential for developing software that maximizes the capabilities of these compact computing platforms while maintaining reliability in often mission-critical environments.
Optimizing microcontroller code for maximum efficiency
Creating efficient code for microcontrollers begins with understanding the fundamental constraints of these platforms. Unlike desktop systems with virtually unlimited resources, microcontrollers typically operate with clock speeds measured in megahertz, memory in kilobytes, and power budgets in milliwatts. Every instruction matters, making optimization not just beneficial but necessary for achieving desired performance targets.
One of the most critical optimization strategies involves minimizing CPU cycles. This means selecting the most efficient algorithms for the task at hand, often favoring simplicity over complexity. For instance, a simple lookup table might outperform a complex mathematical calculation on a microcontroller, despite being less elegant from a purely algorithmic perspective. The goal is to achieve the necessary functionality with the fewest processor cycles possible.
Interrupt handling deserves special attention in microcontroller programming. Poorly designed interrupt service routines (ISRs) can introduce unpredictable timing issues and system instability. Effective interrupt optimization includes keeping ISRs as short as possible, minimizing the time spent with interrupts disabled, and carefully managing shared resources to prevent race conditions. This approach ensures that the system remains responsive while handling external events efficiently.
An optimized microcontroller application isn't merely about making code run faster—it's about creating predictable, deterministic behavior that meets timing constraints while maximizing battery life in portable applications.
Register-level optimization represents another powerful technique for microcontroller code optimization. By understanding the hardware architecture and directly manipulating control registers, developers can achieve significant performance improvements compared to using abstracted library functions. This approach requires deeper hardware knowledge but offers substantial benefits for time-critical operations in real-time systems.
Loop unrolling and function inlining are compiler-level optimizations that can dramatically improve execution speed on microcontrollers. Loop unrolling reduces branch penalties by executing multiple iterations of a loop in sequence, while function inlining eliminates the overhead of function calls by integrating the function's code directly at the call site. These techniques increase code size but can provide substantial performance improvements for time-critical sections of code.
Essential tools for microcontroller software development
Successful microcontroller development relies heavily on having the right toolchain. Unlike general-purpose computers where inefficient code might only cause minor inconvenience, microcontroller applications often have strict timing, power, and reliability requirements that demand specialized development tools. These tools provide the capabilities needed to write, debug, and optimize code for these resource-constrained platforms.
A comprehensive development environment for microcontrollers typically includes a cross-compiler, linker, assembler, debugger, and simulator. Many manufacturers now offer integrated development platforms that combine these tools with device-specific libraries and configuration utilities, streamlining the development process. These specialized environments understand the unique constraints of microcontroller development and provide features specifically designed for embedded systems work.
Static analysis tools play a particularly important role in microcontroller development. These tools examine code without executing it, identifying potential issues like buffer overflows, null pointer dereferences, and memory leaks that could lead to system failures. Given that many microcontroller applications run in safety-critical environments, catching these issues before deployment is invaluable for ensuring system reliability and safety.
Integrated development environments (IDEs)
Modern microcontroller IDEs provide comprehensive project management capabilities alongside powerful code editing features specifically tailored for embedded development. These environments typically offer syntax highlighting for both C/C++ and assembly language, code completion to reduce typing errors, and integrated documentation for microcontroller-specific functions and registers. These features significantly improve developer productivity when working with complex embedded systems.
Manufacturer-specific IDEs like STM32CubeIDE (for STMicroelectronics devices), MPLAB X (for Microchip PIC microcontrollers), and Code Composer Studio (for Texas Instruments devices) offer deep integration with their respective hardware platforms. These tools typically include graphical configuration utilities that simplify the process of setting up complex peripherals, generating initialization code automatically based on visual configurations rather than requiring manual register manipulation.
Cross-platform IDEs such as Eclipse-based environments with appropriate plugins provide flexibility for developers working across multiple microcontroller families. These tools allow teams to maintain consistent development practices even when projects span different hardware architectures. The ability to use a single environment across diverse projects can significantly reduce the learning curve and improve development efficiency.
Debugging probes and analyzers
Hardware debugging tools form an essential component of the microcontroller development toolkit. JTAG and SWD (Serial Wire Debug) interfaces provide direct access to the microcontroller's internal state, allowing developers to set breakpoints, step through code execution, and examine register and memory contents in real-time. These capabilities are crucial for identifying and resolving complex timing and state-dependent issues that might not be apparent through code inspection alone.
Logic analyzers offer visibility into the digital signals flowing between the microcontroller and external components. By capturing and displaying these signals with precise timing information, logic analyzers help developers verify communication protocols, identify timing violations, and debug interactions with sensors, actuators, and other peripheral devices. This visibility is particularly valuable when troubleshooting complex multi-component systems.
Trace capabilities in modern debug probes provide unprecedented insight into program execution. Instruction trace features capture the exact sequence of instructions executed by the processor, enabling developers to reconstruct program flow even after a crash or other unexpected behavior. This powerful capability simplifies the debugging of complex, time-dependent issues that might be difficult to reproduce consistently.
Compilers optimized for microcontrollers
Specialized compilers for microcontrollers go beyond the capabilities of standard compilers by incorporating optimizations specifically designed for embedded systems. These compilers understand the unique constraints and opportunities presented by microcontroller architectures, allowing them to generate code that maximizes performance within tight memory and power budgets.
The GNU Arm Embedded Toolchain represents one of the most widely used compiler collections for ARM Cortex-M based microcontrollers. This open-source toolchain provides robust optimization capabilities and broad platform support, making it a popular choice for both commercial and hobbyist projects. Its wide adoption ensures good community support and regular updates to address emerging requirements.
Commercial compilers from vendors like IAR Systems and Keil offer advanced optimization techniques specifically tuned for microcontroller development. These compilers typically provide more aggressive optimizations than their open-source counterparts, potentially producing smaller and faster code at the expense of licensing costs. For professional development teams working on resource-constrained systems, these performance benefits often justify the investment.
Techniques to minimize memory usage
Memory constraints represent one of the most significant challenges in microcontroller development. With typical devices offering only kilobytes of RAM and limited flash storage, efficient memory utilization becomes critical for implementing complex functionality. Developers must carefully consider both code size (program memory usage) and data memory requirements throughout the development process.
Bitfields and bit manipulation techniques offer substantial memory savings when working with multiple boolean values or small integer quantities. By packing multiple values into a single byte or word, developers can dramatically reduce RAM usage compared to storing each value in a separate variable. This approach is particularly valuable for status flags, configuration options, and other collections of small data items that would otherwise consume disproportionate amounts of memory.
Constant data placement optimization involves storing lookup tables and other read-only data in program memory (flash) rather than RAM. Most microcontrollers provide specific mechanisms for accessing constant data directly from flash, freeing valuable RAM for variables that must be modified during execution. This technique is especially useful for applications that require large tables of pre-calculated values or character strings.
Efficient data structure selection
Choosing appropriate data structures is crucial for optimizing memory usage on microcontrollers. Linked lists, while flexible, introduce significant overhead due to pointer storage requirements - each node typically requires an additional 2-4 bytes for the next pointer. For small collections of items, arrays often provide more memory-efficient storage despite their fixed size limitations.
Specialized data structures like ring buffers (circular buffers) provide efficient FIFO (First-In-First-Out) capabilities with minimal memory overhead. These structures are particularly valuable for buffering data in communication interfaces and sampling systems, allowing temporary storage of data without the overhead of dynamic memory allocation. Their fixed memory footprint makes them well-suited to the predictable behavior required in real-time systems.
Sparse data representations can significantly reduce memory requirements when working with data sets that contain many default or zero values. Instead of storing the entire data set, these techniques store only the non-default values along with their positions. For applications like sensor networks where readings might change infrequently, sparse representations can provide substantial memory savings at the cost of slightly more complex access logic.
Dynamic memory allocation strategies
Many embedded development best practices advise against using dynamic memory allocation (malloc/free) in microcontroller applications due to potential fragmentation and unpredictable timing. However, certain applications benefit from controlled dynamic allocation, particularly when dealing with variable-sized data or complex data structures. In these cases, specialized allocation strategies can mitigate the risks associated with traditional heap management.
Memory pools provide a compromise between static and fully dynamic allocation. By pre-allocating fixed-size blocks at initialization time, memory pools eliminate fragmentation issues while still allowing dynamic behavior. This approach is particularly useful for applications that need to create and destroy objects of consistent sizes, such as network packets or command buffers.
Stack-based allocation offers another alternative for temporary memory needs. By allocating variable-sized arrays on the stack (using C99's variable-length arrays or alloca() where supported), developers can obtain dynamically-sized memory without heap fragmentation concerns. However, this approach requires careful management of stack usage to prevent stack overflow conditions, which typically result in system crashes.
Code size reduction methods
Function factoring represents an effective technique for reducing code size in microcontroller applications. By identifying common code sequences that appear in multiple functions and extracting them into shared utility functions, developers can eliminate duplicate code throughout the application. This approach reduces flash memory usage at the expense of minor performance overhead from the additional function calls.
Compiler optimization flags significantly impact code size and performance. Most compilers for microcontrollers offer specific optimization levels targeting size reduction ( -Os
in GCC-based compilers) or performance enhancement ( -O3
). Understanding these options and selecting appropriate optimization strategies for different parts of the application allows developers to balance size and speed requirements effectively.
Conditional compilation using preprocessor directives enables developers to include or exclude features based on build-time configuration. This capability allows a single codebase to support multiple product variants with different feature sets, including only the code needed for each specific configuration. The result is smaller binaries tailored to each particular device's requirements rather than a one-size-fits-all solution containing unused functionality.
Leveraging Microcontroller-Specific hardware features
Modern microcontrollers incorporate sophisticated peripheral sets that can significantly offload the CPU when properly utilized. Direct Memory Access (DMA) controllers, for instance, can transfer data between memory and peripherals without CPU intervention, allowing the processor to perform other tasks simultaneously. This parallel operation capability can dramatically improve system throughput in data-intensive applications like audio processing or sensor data collection.
Hardware accelerators for cryptography, digital signal processing, and floating-point mathematics provide specialized execution units optimized for specific operations. By leveraging these dedicated hardware resources rather than implementing the same functionality in software, developers can achieve orders of magnitude improvement in both performance and energy efficiency. These accelerators are particularly valuable in applications with intensive computational requirements like encryption, audio processing, or motor control.
Peripheral interconnection systems in advanced microcontrollers allow direct communication between peripherals without CPU involvement. For example, a timer might trigger an ADC conversion, which upon completion triggers a DMA transfer to memory - all without software intervention. This sophisticated capability enables complex, deterministic behaviors while minimizing CPU overhead, leaving processing resources available for application logic rather than hardware management.
The difference between adequate and exceptional microcontroller software often lies in how effectively it leverages hardware-specific features to offload the CPU and minimize power consumption.
Power management features deserve special attention in battery-powered applications. Most modern microcontrollers offer multiple low-power modes that selectively disable peripherals and reduce clock frequencies to minimize energy consumption. By structuring applications to maximize time spent in these low-power states, developers can dramatically extend battery life without sacrificing functionality. This often involves event-driven architectures that allow the processor to sleep until external events require attention.
Hardware timers provide precise timing capabilities critical for many embedded applications. Beyond basic timing functions, advanced timer units can generate complex PWM signals, measure input frequencies and pulse widths, and trigger other peripherals at precise intervals. Understanding and fully utilizing these capabilities allows developers to implement sophisticated timing-dependent behavior with minimal software overhead.
Best practices for robust embedded software
Reliability stands as a paramount concern in microcontroller applications, which often operate in environments where failures can have significant consequences. Building robust embedded software requires disciplined development practices focused on anticipating and handling potential failure modes. This includes comprehensive error detection and recovery mechanisms, careful resource management, and thorough testing under realistic operating conditions.
Watchdog timers provide a hardware-based defense against software lockups and other failure conditions. These specialized timers require periodic "feeding" (resetting) by the application software; failure to reset the watchdog results in a system reset. Properly implementing watchdog handlers ensures that the system can recover automatically from unexpected software failures, providing an additional layer of reliability in deployed systems.
State machine architectures offer significant advantages for microcontroller applications. By decomposing complex behaviors into well-defined states with clear transition conditions, developers can create more predictable and testable software. State machines also simplify error handling by providing natural points for detecting and responding to exceptional conditions, making the system more resilient to unexpected inputs or environmental factors.
Defensive programming techniques
Input validation represents a fundamental defensive programming practice in microcontroller software. By thoroughly checking all inputs—whether from sensors, communication interfaces, or user interactions—before processing them, applications can prevent invalid data from causing unpredictable behavior. This validation should include range checking, format verification, and consistency checks appropriate to each specific input type.
Boundary condition testing focuses specifically on edge cases that often reveal subtle bugs. By explicitly testing behavior at the minimum and maximum allowed values, as well as just beyond these limits, developers can identify and address potential overflow, underflow, and other boundary-related issues before they manifest in deployed systems. This thorough approach to boundary handling is particularly important in safety-critical applications.
Resource monitoring involves tracking system resources like memory usage, stack depth, and processing load during operation. By implementing runtime checks that detect approaching resource limitations, applications can take corrective action before critical failures occur. These mechanisms might include logging warnings when resources reach concerning levels or even gracefully degrading functionality to preserve essential operations.
Error handling mechanisms
Graceful error recovery forms the backbone of robust microcontroller applications. Instead of simply failing when errors occur, well-designed systems implement structured recovery mechanisms that can detect, log, and respond to error conditions without user intervention. This might involve resetting affected subsystems, retrying operations with modified parameters, or falling back to safe operating modes that maintain critical functionality while disabling non-essential features.
Error code standardization significantly improves maintainability in embedded software projects. By establishing consistent error reporting conventions across the codebase, developers can implement generic error handling mechanisms that work uniformly throughout the application. This standardization not only simplifies development but also enhances diagnostic capabilities, allowing for more effective troubleshooting when issues arise in deployed systems.
Fault isolation through modular architecture helps contain the impact of errors when they do occur. By designing systems with clear boundaries between functional components and minimizing shared state, developers can prevent errors in one subsystem from corrupting others. This compartmentalization is particularly valuable in safety-critical applications where partial functionality must be maintained even when components fail.
Effective error handling isn't about preventing all possible errors—it's about ensuring the system can detect, contain, and recover from the inevitable problems that will occur during real-world operation.
Checksums and data validation provide essential protection against memory corruption and communication errors. By attaching validation metadata to critical data structures and communication packets, applications can detect when information has been corrupted and take appropriate recovery actions. These techniques are particularly important in environments with potential for electrical noise, power fluctuations, or radiation effects that might cause bit flips in memory or during data transmission.
Testing embedded software thoroughly
Unit testing in the microcontroller context presents unique challenges compared to desktop software development. The hardware dependencies and resource constraints of embedded systems often make traditional unit testing approaches impractical. However, by using hardware abstraction layers and mock objects to simulate hardware interactions, developers can create effective unit tests that verify individual components without requiring physical hardware for every test case.
Hardware-in-the-loop (HIL) testing represents an essential validation approach for embedded systems. This technique incorporates actual hardware components into the testing environment, allowing software to interact with real sensors, actuators, and communication interfaces rather than simulations. HIL testing catches integration issues and hardware-specific behaviors that might be missed in purely software-based tests, providing confidence that the system will function correctly in its intended environment.
Environmental stress testing evaluates system behavior under extreme conditions. By subjecting hardware to temperature extremes, voltage fluctuations, electromagnetic interference, and mechanical stress while monitoring software behavior, developers can identify weaknesses that might lead to failures in real-world deployments. This testing is particularly important for systems that must operate reliably in harsh industrial environments or safety-critical applications.
Regression testing takes on heightened importance in microcontroller development due to the challenges of updating deployed firmware. Each modification to the codebase should trigger comprehensive regression tests to ensure that existing functionality remains intact. Automated test frameworks that can execute predefined test sequences on target hardware provide an efficient mechanism for performing these tests consistently, reducing the risk of introducing new bugs when fixing existing issues.
Coverage analysis tools help ensure thoroughness in testing embedded applications. By instrumenting code to track which portions execute during testing, these tools identify untested code paths that might harbor latent bugs. Achieving high code coverage, particularly of error handling paths that might rarely execute during normal operation, significantly increases confidence in system reliability before deployment to production environments.
Memory leak detection requires specialized approaches in long-running embedded systems. While traditional leak detection tools might be impractical for resource-constrained devices, strategic placement of memory usage monitoring code at key points in the application lifecycle can help identify gradual resource depletion. This monitoring is particularly critical for systems expected to operate continuously for months or years without rebooting.
Fuzzing techniques, which involve providing invalid, unexpected, or random data to system inputs, have proven highly effective at uncovering vulnerabilities in embedded software. By implementing automated fuzzing of communication interfaces, sensor inputs, and user interactions, developers can discover and address potential failure modes that might otherwise remain hidden until encountered in field operation. This proactive approach to finding edge cases significantly enhances system robustness.