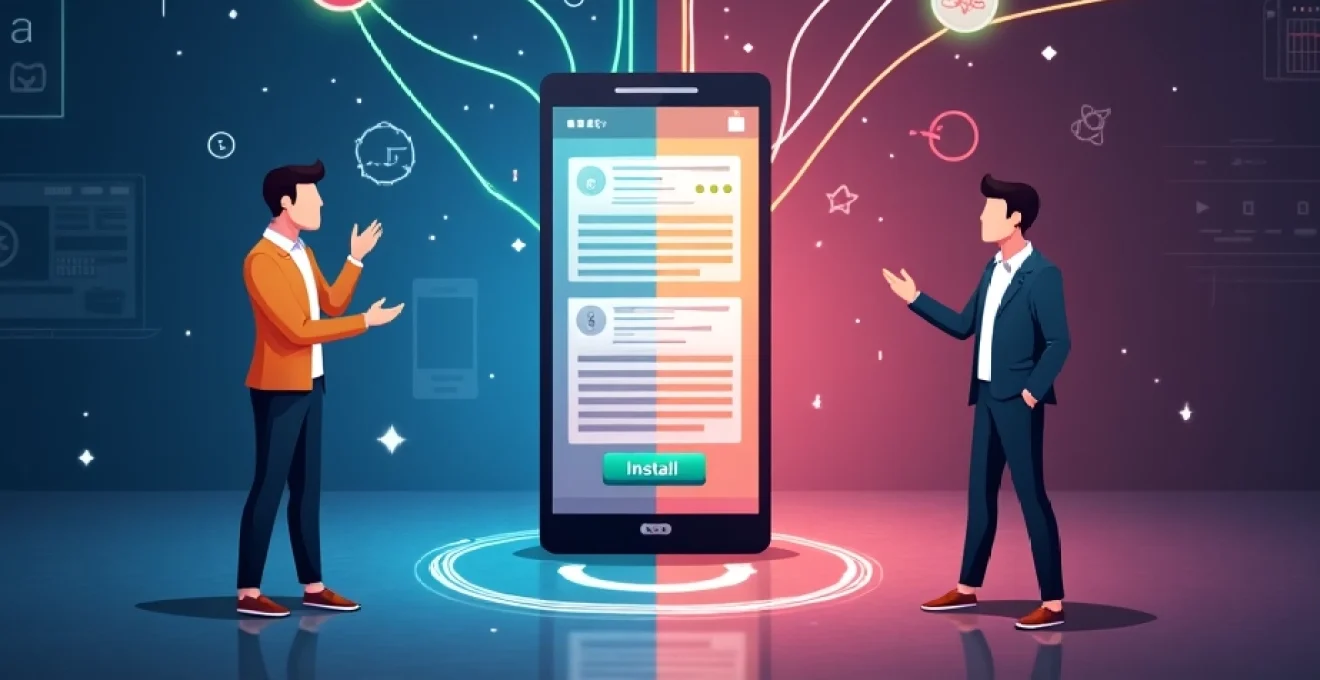
Progressive Web Apps (PWAs) are revolutionizing the way businesses approach web development. By combining the best features of native mobile apps with the accessibility of websites, PWAs offer a powerful solution for enhancing user experience and driving engagement. As mobile internet usage continues to soar, adopting PWA technology can give your site a significant competitive edge. Let's dive into the world of PWAs and explore how they can transform your online presence.
Core principles of progressive web apps (PWAs)
At their core, PWAs are built on three fundamental principles: reliability, speed, and engagement. These principles work together to create a seamless, app-like experience for users directly through their web browsers. PWAs are designed to work offline or in low-quality network conditions, load instantly, and provide an immersive full-screen experience.
Reliability is achieved through service workers, which enable offline functionality and improved performance. Speed is ensured by aggressive caching strategies and optimized loading techniques. Engagement is enhanced through features like push notifications and home screen installation, which keep users coming back to your app.
One of the key advantages of PWAs is their ability to work across all devices and platforms. Unlike native apps that require separate development for iOS and Android, PWAs offer a unified solution that works on any device with a compatible web browser. This cross-platform compatibility significantly reduces development costs and time-to-market.
PWAs represent the future of web development, offering the best of both worlds: the reach of the web and the experience of a native app.
Service workers: enabling offline functionality
Service workers are the backbone of PWAs, enabling offline functionality and improved performance. These JavaScript files run in the background, separate from the web page, and can intercept network requests, cache or retrieve resources from the cache, and enable push notifications. Let's explore some key aspects of service workers:
Caching strategies with workbox
Workbox is a set of libraries and tools that simplify the process of implementing service workers. It provides pre-built caching strategies that you can easily implement in your PWA. Some common caching strategies include:
- Cache-first: Prioritizes serving content from the cache, falling back to the network if necessary.
- Network-first: Attempts to fetch fresh content from the network, using cached content as a fallback.
- Stale-while-revalidate: Serves cached content immediately while fetching updated content in the background.
By implementing these strategies, you can ensure that your PWA remains functional even when users are offline or have a poor internet connection.
Background sync for seamless user experience
Background sync allows PWAs to defer actions until the user has a stable internet connection. This is particularly useful for scenarios like form submissions or data updates. When a user performs an action offline, the service worker can queue the request and automatically retry it when the connection is restored. This ensures that users can continue interacting with your app without worrying about network availability.
Push notifications implementation
Push notifications are a powerful tool for re-engaging users and keeping them informed about updates or new content. Service workers enable PWAs to receive push notifications even when the app is not actively running in the browser. To implement push notifications, you'll need to:
- Request permission from the user to send notifications
- Subscribe the user to your push service
- Store the subscription information on your server
- Send push messages from your server to the user's device
- Handle the incoming push messages in your service worker
Intercepting network requests
Service workers can intercept and modify network requests, allowing you to implement custom caching strategies or serve different content based on the user's network conditions. This capability is crucial for creating a truly offline-first experience. You can use the fetch
event listener in your service worker to intercept requests and decide how to handle them.
Responsive design and Mobile-First approach
Responsive design is a cornerstone of PWA development, ensuring that your app looks and functions well on devices of all sizes. Adopting a mobile-first approach means designing for smaller screens first and then progressively enhancing the experience for larger devices. This strategy aligns perfectly with the progressive enhancement philosophy of PWAs.
Fluid layouts with CSS Grid and Flexbox
CSS Grid and Flexbox are powerful layout tools that enable you to create fluid, responsive designs without relying on media queries for every breakpoint. CSS Grid is particularly useful for creating complex, two-dimensional layouts, while Flexbox excels at managing one-dimensional layouts and alignment.
For example, you can use CSS Grid to create a responsive card layout that automatically adjusts the number of columns based on the available space:
.card-container { display: grid; grid-template-columns: repeat(auto-fit, minmax(250px, 1fr)); gap: 1rem;}
Adaptive images using srcset and sizes
Optimizing images for different screen sizes and resolutions is crucial for improving loading times and conserving bandwidth. The srcset
and sizes
attributes allow you to provide multiple image sources and let the browser choose the most appropriate one based on the device's characteristics and viewport size.
Touch-friendly UI components
Creating touch-friendly UI components is essential for providing a good user experience on mobile devices. Some key considerations include:
- Using appropriate touch target sizes (at least 48x48 pixels)
- Implementing touch gestures for common actions (e.g., swipe to refresh)
- Providing visual feedback for touch interactions
By focusing on these aspects of responsive design and mobile-first development, you can ensure that your PWA provides an excellent user experience across all devices.
App manifest and installation process
The Web App Manifest is a JSON file that provides information about your PWA, enabling it to be installed on a user's device and behave like a native app. This manifest file is crucial for creating a seamless installation experience and defining how your app should appear when launched.
Configuring the web app manifest JSON
The Web App Manifest includes several key properties that define your PWA's behavior and appearance:
Property | Description |
---|---|
name | The full name of your PWA |
short_name | A shorter name for use on home screens |
icons | An array of icon objects for different sizes and resolutions |
start_url | The URL that should be loaded when the app is launched |
display | The preferred display mode (e.g., "standalone", "fullscreen") |
theme_color | The color of the app's toolbar |
background_color | The background color of the splash screen |
Add to home screen (A2HS) implementation
The Add to Home Screen (A2HS) feature allows users to install your PWA directly from the browser, creating a shortcut on their device's home screen. To enable A2HS, you need to meet certain criteria:
- Have a valid Web App Manifest
- Serve your app over HTTPS
- Register a service worker with a fetch event handler
- Meet the browser's engagement heuristics (e.g., repeated visits)
Once these criteria are met, most browsers will automatically prompt users to install your PWA. However, you can also implement custom install prompts for more control over the installation experience.
Custom install prompts with beforeinstallprompt event
The beforeinstallprompt
event allows you to create a custom installation flow for your PWA. By listening for this event, you can defer the browser's native install prompt and instead show your own UI to guide users through the installation process.
Here's a basic example of how to implement a custom install prompt:
let deferredPrompt;window.addEventListener('beforeinstallprompt', (e) => { e.preventDefault(); deferredPrompt = e; showInstallPrompt();});function showInstallPrompt() { // Show your custom install UI here}function installPWA() { deferredPrompt.prompt(); deferredPrompt.userChoice.then((choiceResult) => { if (choiceResult.outcome === 'accepted') { console.log('User accepted the install prompt'); } deferredPrompt = null; });}
By implementing custom install prompts, you can create a more tailored and engaging installation experience for your users, potentially increasing the adoption rate of your PWA.
Performance optimization techniques for PWAs
Performance is a critical factor in the success of any web application, and PWAs are no exception. In fact, one of the key advantages of PWAs is their ability to deliver fast, app-like experiences even on slower networks or less powerful devices. Let's explore some essential performance optimization techniques for PWAs:
Lighthouse audits for PWA scoring
Lighthouse is an open-source tool developed by Google that audits web pages and PWAs for performance, accessibility, and best practices. It provides a PWA score based on several criteria, including:
- Fast and reliable network performance
- Installability
- PWA optimized
- HTTPS implementation
Running Lighthouse audits regularly can help you identify areas for improvement and ensure that your PWA meets the highest standards of performance and user experience.
Code splitting with Webpack
Code splitting is a technique that involves breaking your application into smaller chunks that can be loaded on demand. This approach can significantly improve initial load times by reducing the amount of JavaScript that needs to be downloaded and parsed before the app becomes interactive.
Webpack, a popular module bundler, provides built-in support for code splitting. You can use dynamic imports to split your code into smaller chunks:
import('./module').then(module => { // Use the module here});
By implementing code splitting, you can ensure that users only download the code they need for the current route or feature, leading to faster load times and improved performance.
Lazy loading with intersection Observer API
Lazy loading is a technique that defers the loading of non-critical resources until they are needed. This can significantly improve initial page load times and conserve bandwidth. The Intersection Observer API provides an efficient way to implement lazy loading for images and other content.
Here's a basic example of how to implement lazy loading for images using the Intersection Observer API:
const observer = new IntersectionObserver((entries) => { entries.forEach(entry => { if (entry.isIntersecting) { const img = entry.target; img.src = img.dataset.src; observer.unobserve(img); } });});document.querySelectorAll('img[data-src]').forEach(img => { observer.observe(img);});
PRPL pattern implementation
The PRPL pattern (Push, Render, Pre-cache, Lazy-load) is a performance optimization strategy specifically designed for PWAs. It focuses on optimizing the initial load and subsequent navigation experiences. Here's how to implement the PRPL pattern:
- Push critical resources for the initial route
- Render initial route as quickly as possible
- Pre-cache remaining routes
- Lazy-load other routes and non-critical assets
By following the PRPL pattern, you can ensure that your PWA loads quickly and provides a smooth, app-like experience for users.
PWA frameworks and tools
Several frameworks and tools are available to simplify the process of building and optimizing PWAs. Let's explore some popular options:
Developing with Google's Workbox
Workbox is a set of libraries and tools developed by Google to make it easier to build high-quality PWAs. It provides a range of features, including:
- Pre-configured caching strategies
- Background sync
- Offline analytics
- Workbox CLI for generating service worker code
Workbox simplifies many of the complex tasks involved in PWA development, allowing you to focus on creating great user experiences.
Vue.js and Nuxt.js for PWA creation
Vue.js is a popular JavaScript framework for building user interfaces, and Nuxt.js is a higher-level framework built on top of Vue.js that provides additional features and optimizations. Both of these frameworks offer excellent support for PWA development.
Nuxt.js, in particular, provides a PWA module that automatically generates a service worker and handles many PWA-related tasks out of the box. This can significantly speed up the development process and ensure that your PWA adheres to best practices.
React-based PWAs with Create React App
Create React App is a popular toolchain for building React applications, and it includes built-in support for PWAs. When you create a new project with Create React App, you can opt-in to PWA features by registering a service worker in your index.js
file:
import * as serviceWorkerRegistration from './serviceWorkerRegistration';serviceWorkerRegistration.register();
This will enable offline capabilities, caching, and other PWA features in your React application.
Angular service worker module
Angular, another popular framework for building web applications, provides a built-in service worker module that makes it easy to add PWA capabilities to your Angular apps. The Angular service worker is preconfigured to handle common PWA tasks, such as:
- Caching application resources
- Updating cached resources when new versions are available
- Serving cached resources when offline
- Handling push notifications
By leveraging these frameworks and tools, you can streamline the process of building and optimizing your PWA, ensuring that you deliver a high-quality, performant experience to your users.
Adopting PWA technology can significantly enhance your site's performance, user engagement, and overall success in today's mobile-first digital landscape.
As you embark on your PWA development journey, remember that the key to success lies in focusing on user experience, performance optimization, and continuous improvement. By leveraging the power of service workers, responsive design, and performance optimization techniques, you can create a PWA that not only meets but exceeds user expectations.